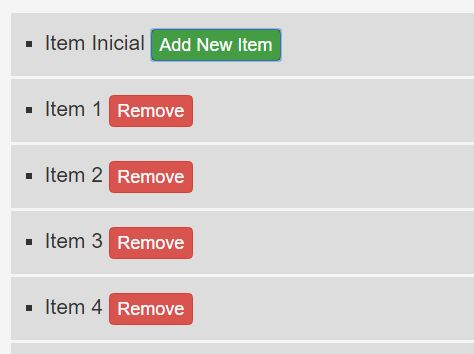
This is my third post related to problem-solving (helping other engineers). If you want to see my last post, you can click on the link https://lnkd.in/dheqUD7E
So I pick another small problem with a large impact: Adding a new line item in a DOM.
There are easier ways in jQuery and other libraries to solve this problem, but I am using vanilla JavaScript for the sake of a better understanding for devs.
In addition, I’ve added CSS style, if you copy and paste the below-mentioned code into your IDE, you will be able to see a better user experience.
<style>
hashtag#description {
height: 40px;
border: 1px solid hashtag#ddd;
box-shadow: 0 2px 10px 0px hashtag#ddd;
padding: 5px;
box-sizing: border-box;
border-radius: 4px;
}
hashtag#add {
height: 40px;
background-color: hashtag#ff821f;
color: hashtag#fff;
font-weight: bold;
border: 1px solid hashtag#ff821f;
border-radius: 4px;
box-shadow: 0 2px 10px 0px hashtag#ddd;
}
hashtag#item-container {
list-style-type: none;
padding: 0;
}
hashtag#item-container li {
background-color: hashtag#eee;
margin-bottom: 5px;
padding: 5px;
box-shadow: 0 2px 10px 0px hashtag#eee;
border: 1px solid hashtag#ff821f;
border-radius: 4px;
}
</style>
<input type="text" id='description' >
<button onclick='addNewElementInDom()' id="add">Add New Element</button>
<ul id="item-container"></ul>
<script>
const addNewElementInDom = () => {
const inputElement = document.getElementById('description');
const newElement = document.createElement('li');
const itemContainer = document.getElementById('item-container');
newElement.textContent = inputElement.value;
if(inputElement.value) {
itemContainer.appendChild(newElement);
inputElement.value = null;
}
return
}
</script>