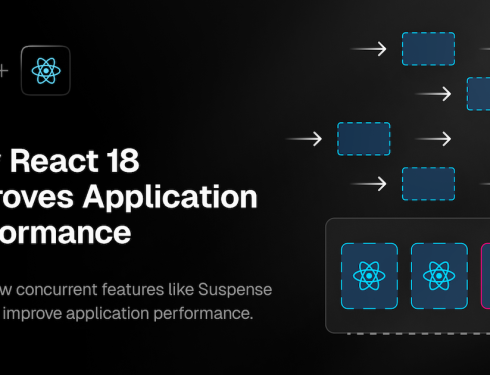
While React 18 calls useEffect twice during the initial render, this does not mean you should stop using useEffect for API calls. It is still a useful tool, however, here are some ideas for dealing with potential concerns and other approaches:
1. Look for a flag or state variable to ensure the API call is only executed once.
useEffect(() => {
if (!hasFetchedData) {
fetchData();
setHasFetchedData(true);
}
}, []);
2. Consider Suspense for Data Collection:
– Use Suspense and React.lazy to declaratively mark components that require data.
– React handles loading and error states, potentially simplifying API handling.
– Wrap data-required components in <Suspense> boundaries.
– Use fetch to retrieve data with automatic loading state management.
Note: I haven’t upgraded my React project to React 18, all the above tips are gathered from research.